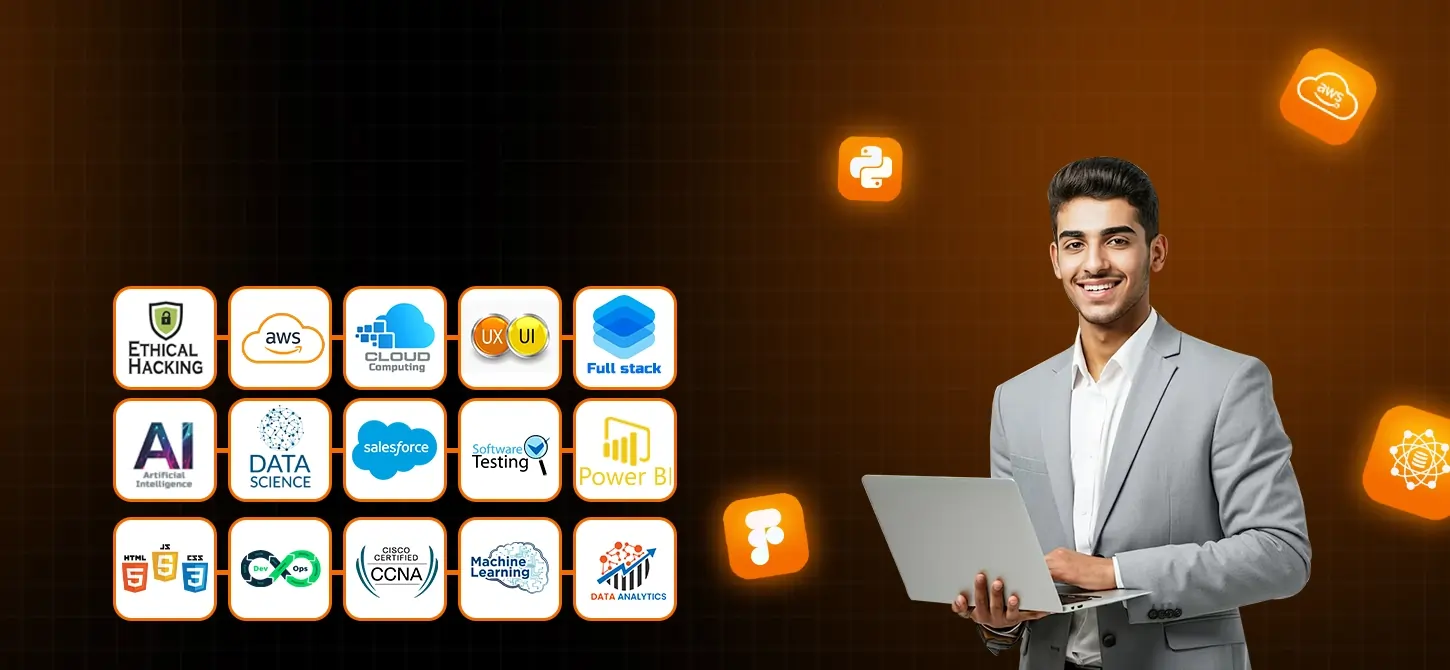
Your Dream Job Awaits
- Let's Make It Happen
"SevenMentor, a leading educational institute, has transformed countless careers with its industry-focused courses. Now, it's your turn to take the leap. Unlock your potential and build the career you've always dreamed of let's make it happen!"
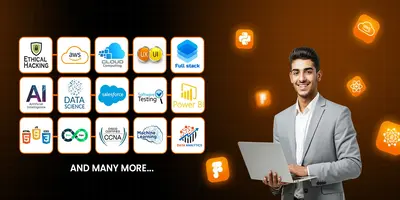
Behind Every Successful Career
Sevenmentor – A Renowned Brand For Quality Education And In-house Job Placement Services Since Past Decades.
0+
Offline/Online Courses
0+
Classrooms
0+
Total Mentors
0+
Successful Careers Made
One Platform, Infinite Possibilities.
Empower Your Future with Our Industry-Focused Professional Programs
Unlock your potential with expert-led, career-focused training that prepares you for the real world. At SevenMentor, we offer immersive, industry-aligned learning designed to build the skills top companies seek. Gain hands-on experience, learn from seasoned mentors, and take your next big career step with confidence.
The future you want starts here.
OUR COURSES
Programs To Help You Upskill which Lands you to your DREAM JOB
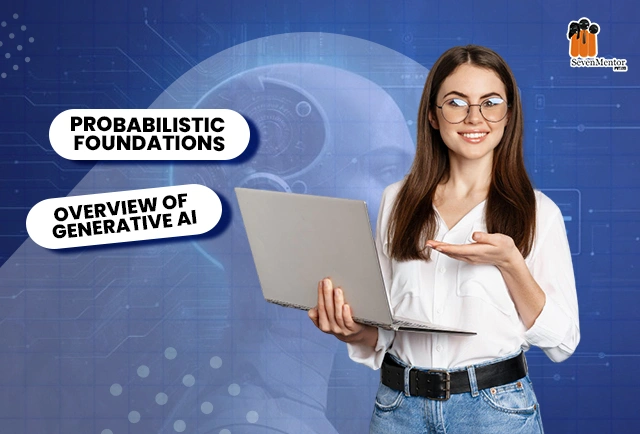
Advance Generative AI
Explore the cutting edge of AI with advanced generative models and learn to create intelligent systems that produce text, images, code, and more—reshaping the future of technology and innovation.
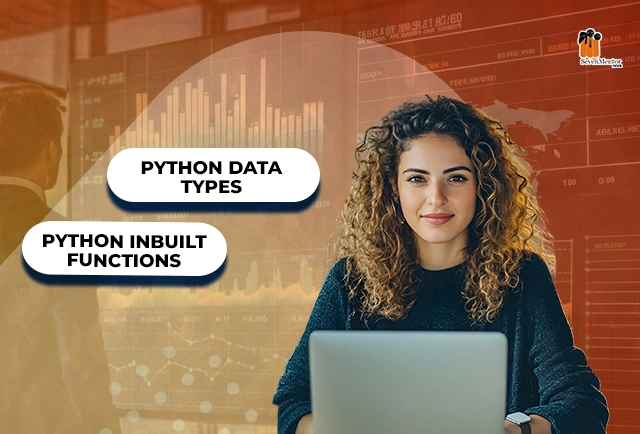
Data Analytics
Master data storytelling and visualization techniques using Data Analytics.
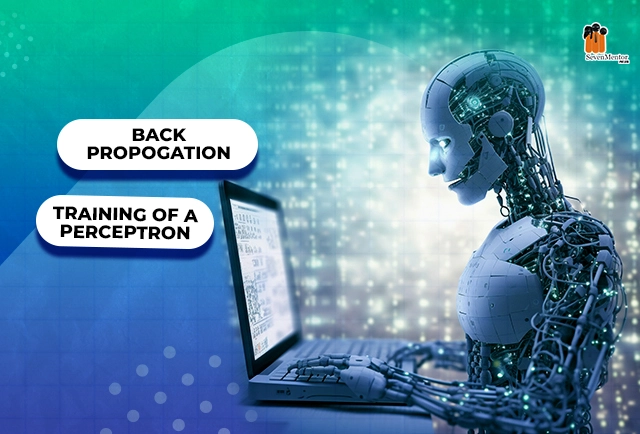
Artificial Intelligence
Deep dive into Artificial Intelligence algorithms, neural networks, and practical AI applications.
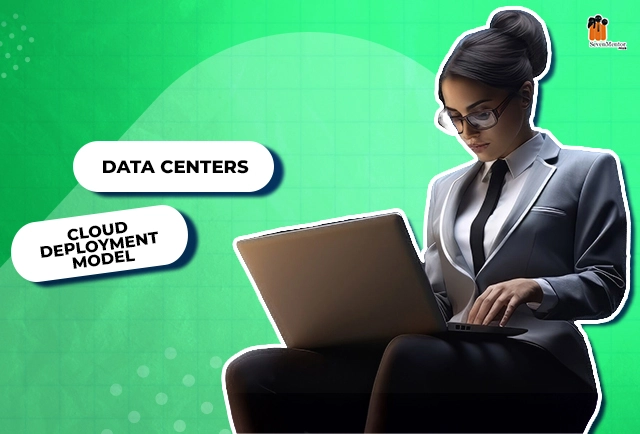
Amazon Web Services
Understand Amazon Web Services technologies.
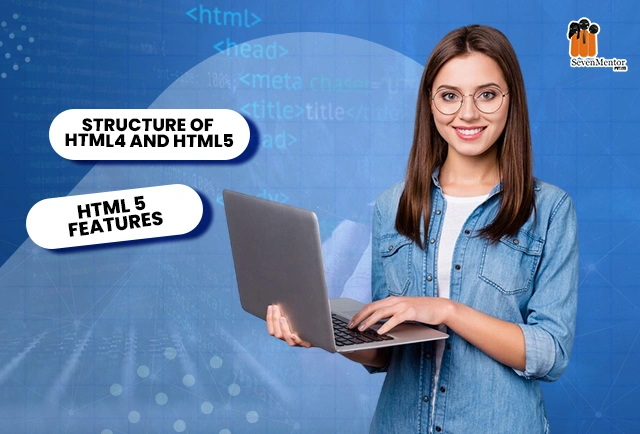
Full Stack With Python
Learn to build dynamic, end-to-end web applications using Python—from frontend design to backend development—and launch your career as a versatile full stack developer.
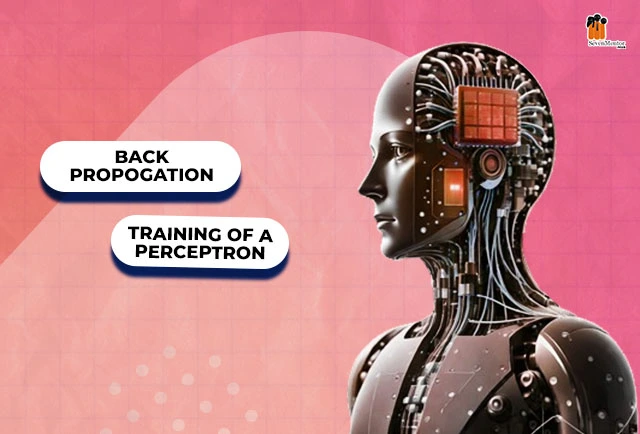
Machine Learning
Learn deep learning techniques and implement neural networks with Machine Learning.
INDUSTRY AFFILIATIONS
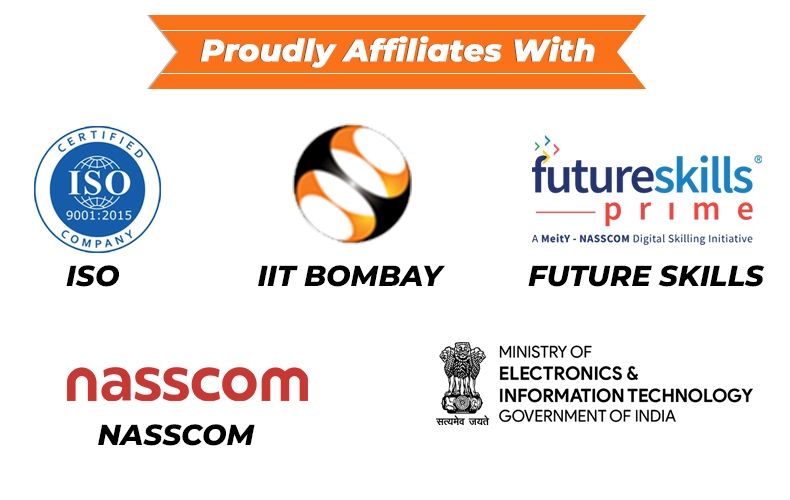
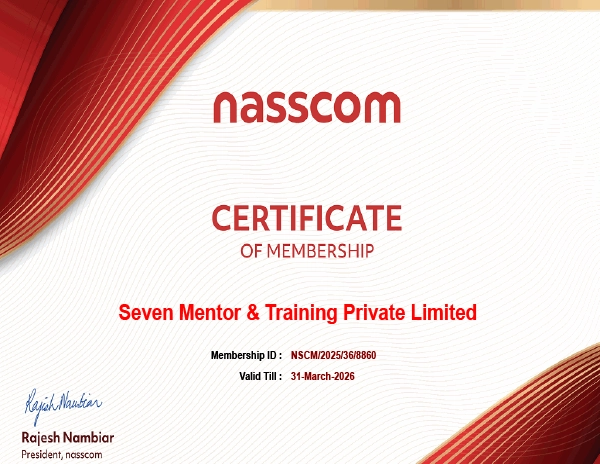
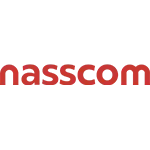
NASSCOM CERTIFICATION
Get industry-recognized certification backed by NASSCOM's excellence.
About This Partnership
NASSCOM is India's premier industry association for IT and business process management. Our partnership with NASSCOM ensures that our curriculum meets industry standards and provides students with credentials recognized by top employers nationwide. This affiliation validates the quality and relevance of our training programs in the ever-evolving tech landscape.
PROFESSIONAL CERTIFICATIONS

ISO CERTIFICATION
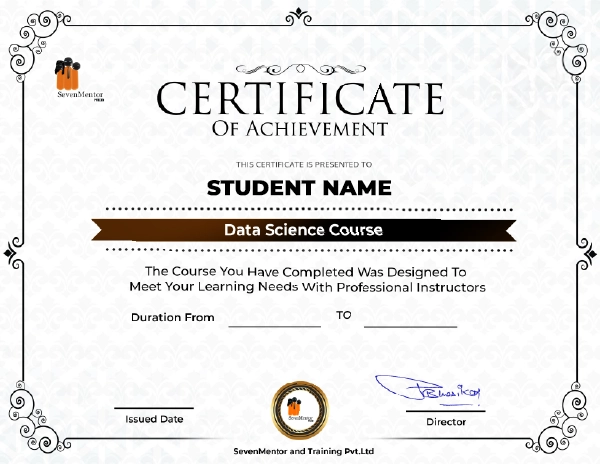
Validate your skills with an internationally recognized ISO certification.

IIT BOMBAY SPOKEN TUTORIAL CERTIFICATE
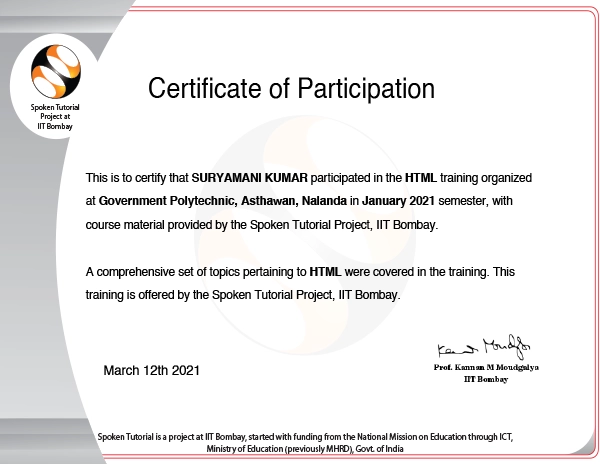
Earn a prestigious certification from one of India's premier institutions.

QUIZ CERTIFICATE
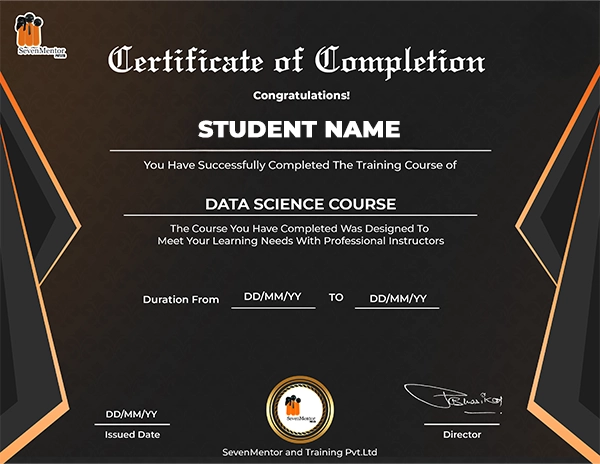
Earn a prestigious certification from one of India's premier institutions.
Our Collaboration
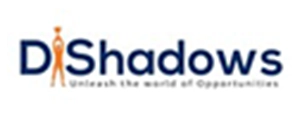
DiShadows Corporation
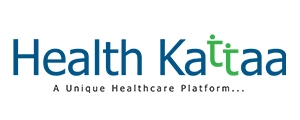
Health Kattaa
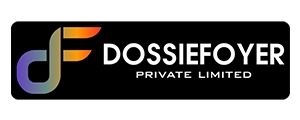
Dossiefoyer Pvt. Ltd.
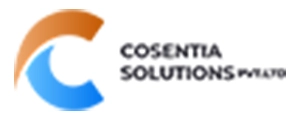
Cosentia Solutions Pvt. Ltd.
Why Should You Upskill Now?
Join Seven Mentor - Pune's Premier Training Institute for a Future-Ready Career
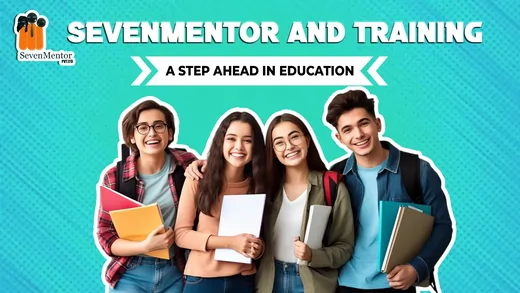
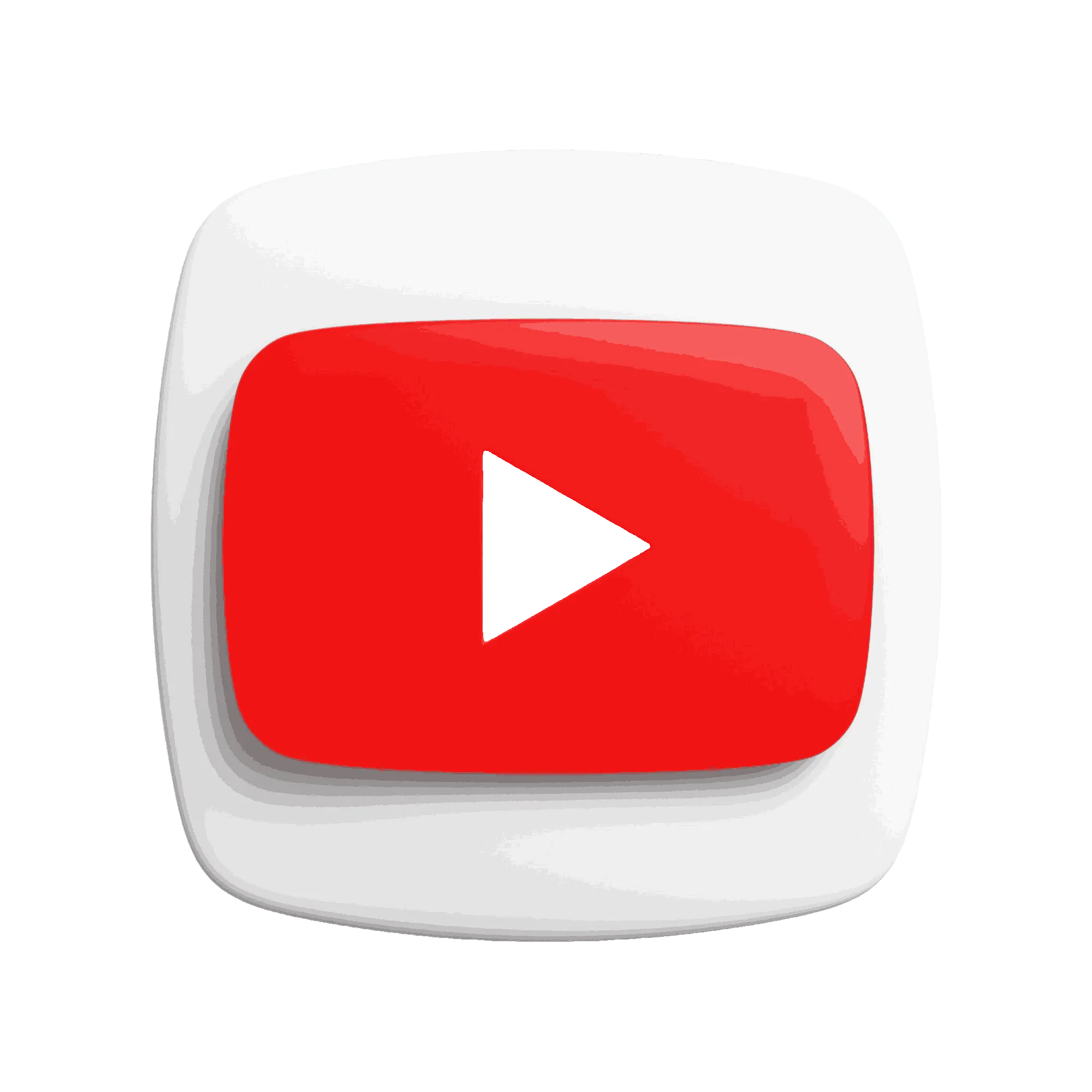
Celebrating Excellence: A Legacy of Awards
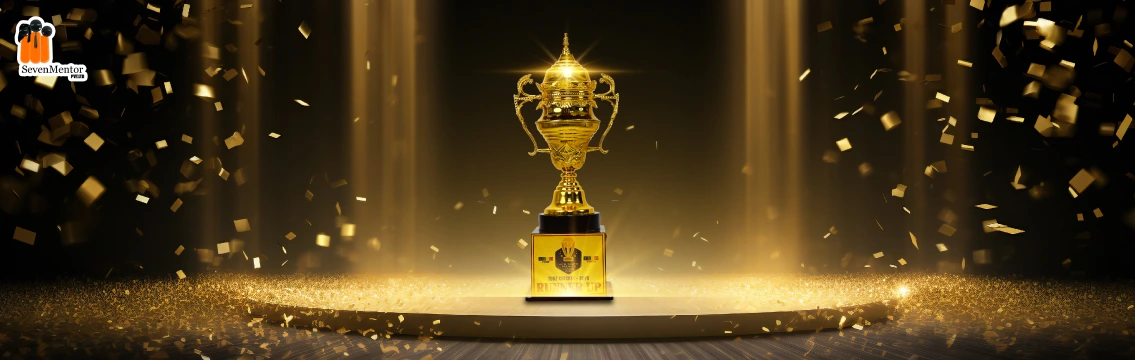
2023 Winner
For IT & Technical Training
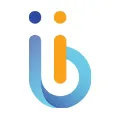
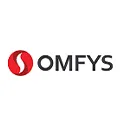
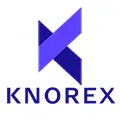
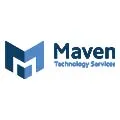
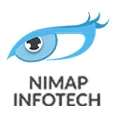
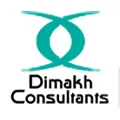
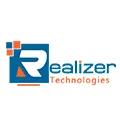
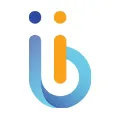
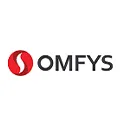
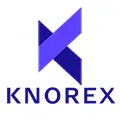
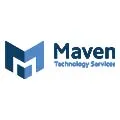
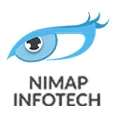
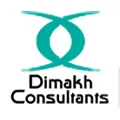
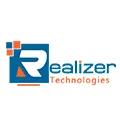
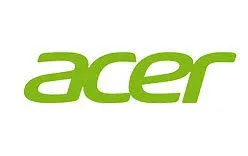
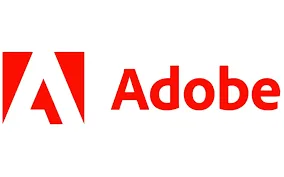
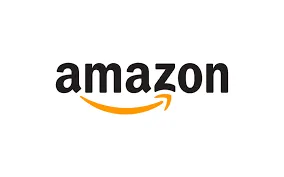
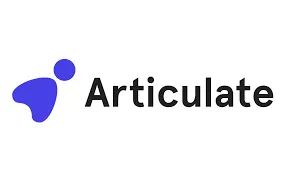
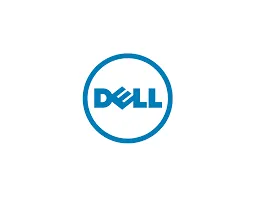
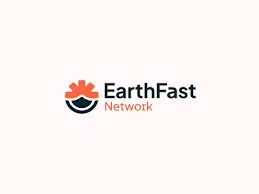
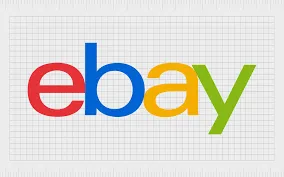
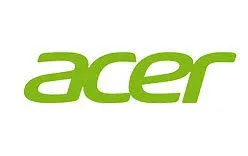
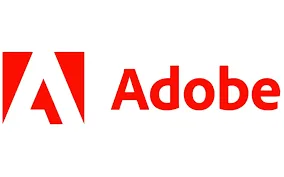
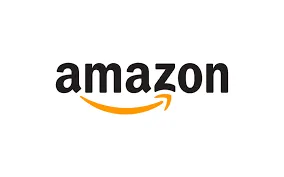
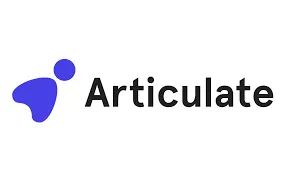
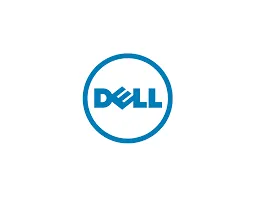
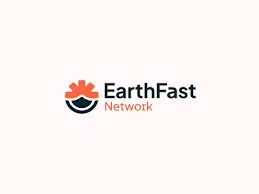
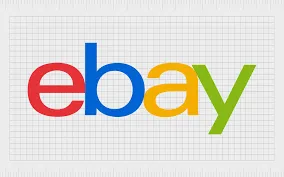
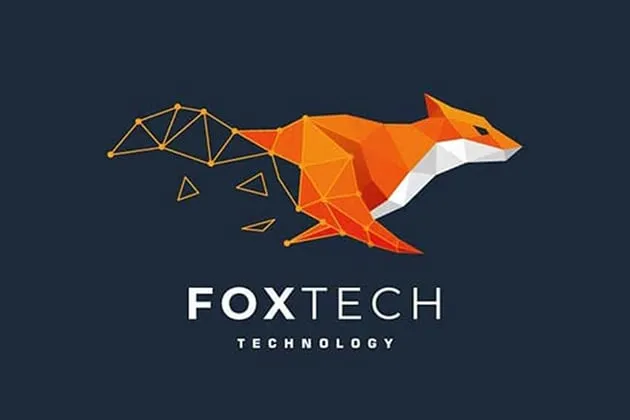
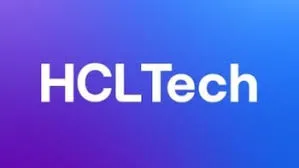
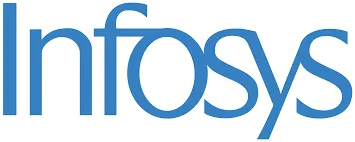
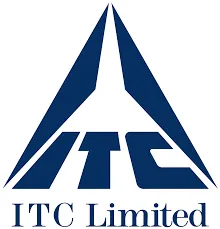
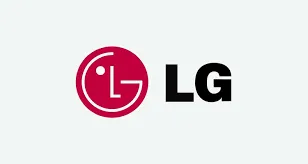
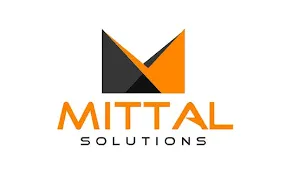
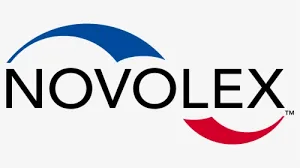
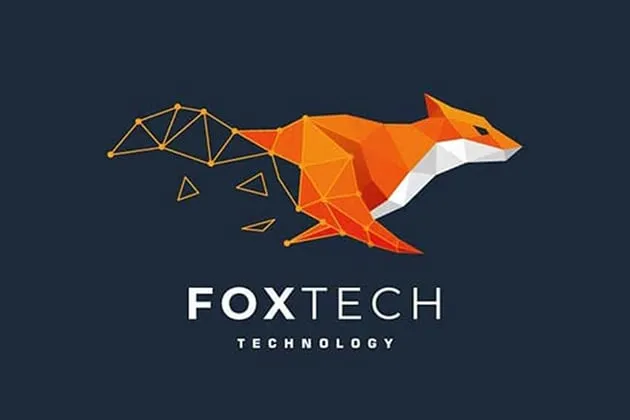
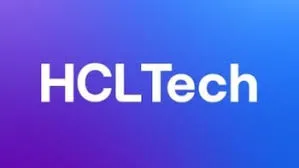
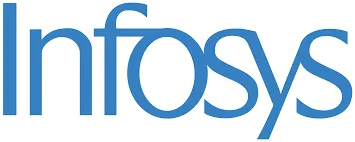
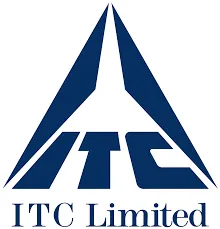
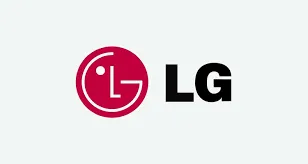
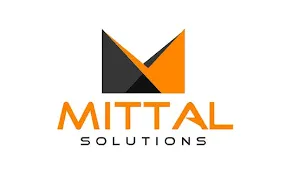
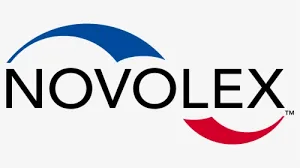
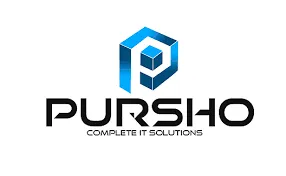
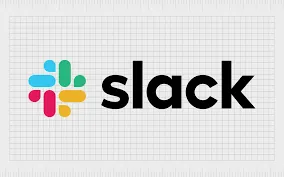
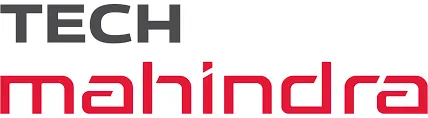
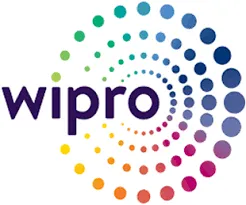
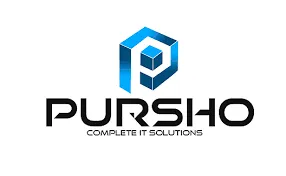
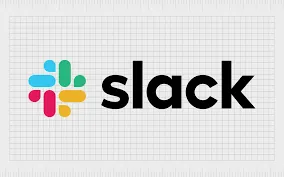
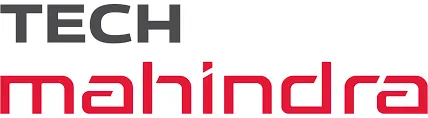
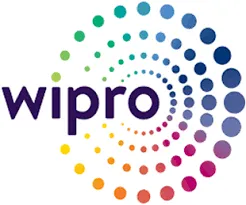
Our Success Lies In Our Learner's, Success Stories
Explore our student's experiences and discover how we've helped them achieve their goals through excellence and dedication.